Authentication payment page integration
The authentication payment page is designed to automate the authentication process. Once payment authentication is
completed, merchants can proceed with payment authorization at any time.
Enter PAYER_AUTH in the transaction_type field to invoke the authentication payment page. Please refer to the JavaScript SDK for other payment page options.
After completing the authentication on the authentication payment page, you can call back the payment authorization API to authorize the payment in two ways.
-
PAYMENT_PA
: After the payment authorization, the capture will be automatically processed.
-
AUTHORIZE_PA
: In this model, only payment authorization will be performed. The acquiring process will either be handled through the manual acquiring API or processed directly by the merchant.
** An additional contract is required for the manual acquiring API.
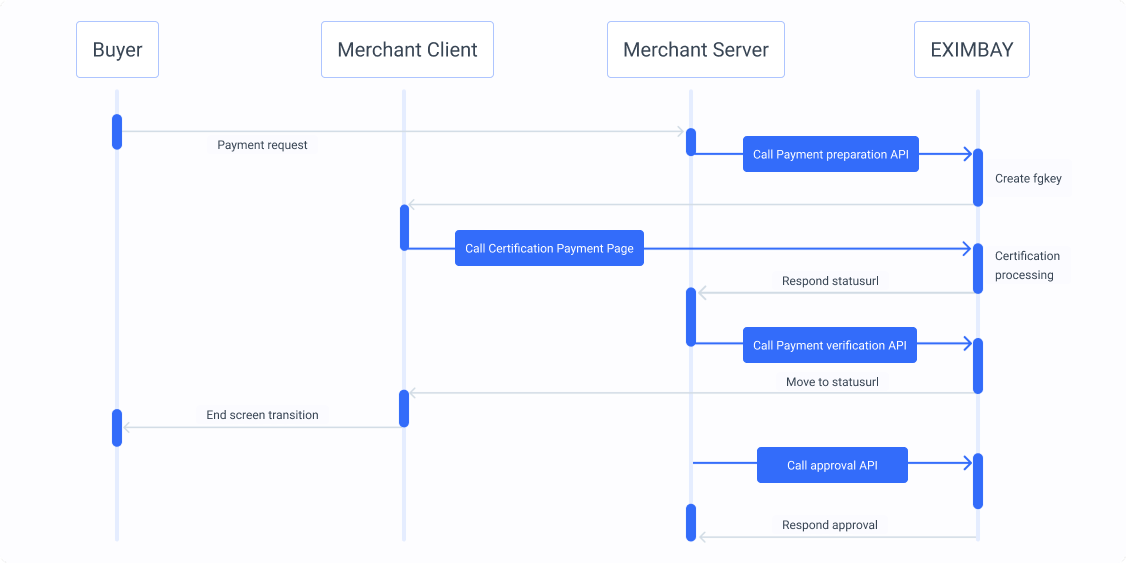
1. SDK Preparation
Kindly add the Eximbay JavaScript SDK library to the HTML page to integrate the payment page.
<!-- jQuery -->
<script type="text/javascript" src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<!-- SDK -->
<script type="text/javascript" src="https://api-test.eximbay.com/v1/javascriptSDK.js"></script>
After installing the Eximbay JavaScript SDK library, you can invoke the EXIMBAY.request_pay() method to initiate the payment page.
For detailed information about the SDK, please refer to the JavaScript SDK documentation
2. Payment Preparation
Eximbay validates parameters for fraud prevention. Please generate an FGKey using the Payment Preparation API to initiate the payment page.
For instructions on generating an FGKey, please refer to the Payment Preparation documentation.
curl --request POST 'https://api-test.eximbay.com/v1/payments/ready' \
--header 'Authorization: Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=' \
--header 'Content-Type: application/json' \
--data '{
"payment" : {
"transaction_type" : "PAYER_AUTH",
"order_id" : "20220819105102",
"currency" : "USD",
"amount" : "1",
"lang" : "EN"
},
"merchant" : {
"mid" : "1849705C64"
},
"buyer" : {
"name" : "eximbay",
"email" : "test@eximbay.com"
},
"url" : {
"return_url" : "eximbay.com",
"status_url" : "eximbay.com"
}
}'
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Type", "application/json");
headers.add("Authorization", "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=");
URI url = URI.create("https://api-test.eximbay.com/v1/payments/ready");
String body = "{\n" +
"\"payment\" : {\n" +
"\"transaction_type\" : \"PAYER_AUTH\",\n" +
"\"order_id\" : \"20220819105102\",\n" +
"\"currency\" : \"USD\",\n" +
"\"amount\" : \"1\",\n" +
"\"lang\" : \"EN\"\n" +
"},\n" +
"\"merchant\" : {\n" +
"\"mid\" : \"1849705C64\"\n" +
"},\n" +
"\"buyer\" : {\n" +
"\"name\" : \"eximbay\",\n" +
"\"email\" : \"test@eximbay.com\"\n" +
"},\n" +
"\"url\" : {\n" +
"\"return_url\" : \"eximbay.com\",\n" +
"\"status_url\" : \"eximbay.com\"\n" +
" }\n" +
"}";
HttpEntity<String> entity = new HttpEntity<>(body, headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.POST, entity, String.class);
System.out.println(response.getBody());
<?php
$url = 'https://api-test.eximbay.com/v1/payments/ready';
$data = '{
"payment": {
"transaction_type": "PAYER_AUTH",
"order_id": "20220819105102",
"currency": "USD",
"amount": "1",
"lang": "EN"
},
"merchant": {
"mid": "1849705C64"
},
"buyer": {
"name": "eximbay",
"email": "test@eximbay.com"
},
"url": {
"return_url": "eximbay.com",
"status_url": "eximbay.com"
}
}';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json', 'Authorization: Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo='));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
echo $response;
curl_close($ch);
?>
import requests
import json
url = "https://api-test.eximbay.com/v1/payments/ready"
headers = {
"Authorization": "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=",
"Content-Type": "application/json"
}
request = {
"payment": {
"transaction_type": "PAYER_AUTH",
"order_id": "20220819105102",
"currnecy": "USD",
"amount": "1",
"lang": "EN"
},
"merchant": {
"mid": "1849705C64",
},
"buyer": {
"name": "eximbay",
"email": "test@eximbay.com"
},
"url": {
"return_url": "eximbay.com",
"status_url": "eximbay.com"
}
}
response = requests.post(url, headers=headers, data=json.dumps(request))
print(response.text)
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api-test.eximbay.com/v1/payments/ready',
'headers': {
'Authorization': 'Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=',
'Content-Type': 'application/json'
},
body: JSON.stringify({
"payment": {
"transaction_type": "PAYER_AUTH",
"order_id": "20220819105102",
"currency": "USD",
"amount": "1",
"lang": "EN"
},
"merchant": {
"mid": "1849705C64"
},
"buyer": {
"name": "eximbay",
"email": "test@eximbay.com"
},
"url": {
"return_url": "eximbay.com",
"status_url": "eximbay.com"
}
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
string uri = "https://api-test.eximbay.com/v1/payments/ready";
string body = "{\n" +
"\"payment\" : {\n" +
"\"transaction_type\" : \"PAYER_AUTH\",\n" +
"\"order_id\" : \"20220819105102\",\n" +
"\"currency\" : \"USD\",\n" +
"\"amount\" : \"1\",\n" +
"\"lang\" : \"EN\"\n" +
"},\n" +
"\"merchant\" : {\n" +
"\"mid\" : \"1849705C64\"\n" +
"},\n" +
"\"url\" : {\n" +
"\"return_url\" : \"eximbay.com\",\n" +
"\"status_url\" : \"eximbay.com\"\n" +
" }\n" +
"}";
WebClient webClient = new WebClient();
webClient.Headers[HttpRequestHeader.ContentType] = "application/json";
webClient.Headers[HttpRequestHeader.Authorization] = "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=";
webClient.Encoding = UTF8Encoding.UTF8;
string responseJSON = webClient.UploadString(uri, body);
Console.Write(responseJSON);
val restTemplate = RestTemplate()
val headers = HttpHeaders()
headers.add("Content-Type", "application/json")
headers.add("Authorization", "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=")
var url = URI.create("https://api-test.eximbay.com/v1/payments/ready")
val body = "{\n" +
"\"payment\" : {\n" +
"\"transaction_type\" : \"PAYER_AUTH\",\n" +
"\"order_id\" : \"20220819105102\",\n" +
"\"currency\" : \"USD\",\n" +
"\"amount\" : \"1\",\n" +
"\"lang\" : \"EN\"\n" +
"},\n" +
"\"merchant\" : {\n" +
"\"mid\" : \"1849705C64\"\n" +
"},\n" +
"\"buyer\" : {\n" +
"\"name\" : \"eximbay\",\n" +
"\"email\" : \"test@eximbay.com\"\n" +
"},\n" +
"\"url\" : {\n" +
"\"return_url\" : \"eximbay.com\",\n" +
"\"status_url\" : \"eximbay.com\"\n" +
" }\n" +
"}"
var entity = HttpEntity<String>(body, headers)
var response = restTemplate.exchange(url, HttpMethod.POST, entity, String::class.java)
println(response.body)
The response value is provided in the fgkey field.
{
"rescode": "0000",
"resmsg": "Success",
"fgkey": "0E9BE04BA239A519E68171F26B68604ADA0A85C8350DBF5C8C0FCCF98461DB09"
}
3. Payment Page Initiation
After generating the fgkey through the payment preparation API, use the response value to initiate the SDK process.
Enter PAYER_AUTH in the transation_type field to invoke the Integrated Payment Page.
When using the SDK to initiate the payment page, ensure that the request parameters align with those of the previously initiated Payment Preparation API. Any discrepancies in parameter values may result in an fgkey mismatch, leading to payment failure.
<script type="text/javascript" src="https://api-test.eximbay.com/v1/javascriptSDK.js"></script>
After installing the SDK library, you may initiate the request_pay method using the EXIMBAY object. If the payment page successfully pops up, the integration is complete.
<button type="button" onclick="payment();">Payment page integration</button>
.
.
.
<script type="text/javascript">
function payment() {
EXIMBAY.request_pay({
"fgkey" : "0E9BE04BA239A519E68171F26B68604ADA0A85C8350DBF5C8C0FCCF98461DB09"
"payment" : {
"transaction_type" : "PAYER_AUTH",
"order_id" : "20220819105102",
"currency" : "USD",
"amount" : "1",
"lang" : "EN"
},
"merchant" : {
"mid" : "1849705C64"
},
"buyer" : {
"name" : "eximbay",
"email" : "test@eximbay.com"
},
"url" : {
"return_url" : "eximbay.com",
"status_url" : "eximbay.com"
}
});
}
</script>
Payment Information Parameters
Required Parameters to Initiate the Payment Page.
fgkey string
Mandatorypayment object
transaction_type string
Mandatory-
PAYER_AUTH
: In this model, only verification will be performed during the payment page initiation. Automated authorization and acquiring API or authorization-only API is required for this process.
* An additional contract is required for the manual acquiring API.
order_id string
MandatoryThis is a unique identifier used to classify a merchant's order. This value cannot be reused for failed orders.
currency string
MandatoryPayment Currency Unit. For detailed information, please refer to the currency code.
amount string
MandatoryTotal Payment Amount. The amount must be greater than "0" and , cannot be used.
lang string
MandatoryLanguage Code: Specifies the language used on the payment page.
payment_method string
Payment Method Code: Defines the designated payment method that will redirect to the payment page. Please refer to the payment method code for details.
multi_payment_method string
* For Cross-Border payment Integration.
merchant object
mid string
MandatoryA unique merchant ID generated by Eximbay to identify the merchant.
shop string
Store Name: If the store name differs from the merchant name, please specify accordingly.
partner_code string
Partner code
url object
return_url string
MandatoryURL Information for the Merchant Page which where the buyer will be redirected after completing the payment process and receiving the payment result. The return URL is browser-based for the customer. If the browser is forcefully terminated, the return URL will not be initiated.
status_url string
Mandatory* Database tasks and payment processing should be handled within the statusURL. The returnURL will not be invoked if the customer forcefully terminates the session
* Duplicate invocation is allowed; however, please ensure that the payment is not processed multiple times.
buyer object
name string
MandatoryCustomer's name
phone_number string
Customer's contact number
email string
MandatoryCustomer's email address. (for sending payment confirmation letter)
tax object* This parameter is used for Korean local payment processing.
receipt_status string
Select Y or N for issuing a cash payment receipt. For bank transfer payments, you must choose either Y or N for receipt issuance.
amount_tax_free string
The amount deducted for tax from the total payment.
amount_taxable string
The amount for tax from the total payment.
amount_vat string
The amount for VAT from the total payment.
amount_service_fee string
The amount for service charge from the total payment.
Note. 1 - All parameters in the tax object are mandatory for Naver Point payments.
other_param object
param1 string
A preparatory parameter available for merchants if needed. The parameter length is limited to 255 characters.
param2 string
A preparatory parameter available for merchants if needed. The parameter length is limited to 255 characters.
product array * The array length is limited to three.
name string
MandatoryMerchandise Name
quantity string
MandatoryMerchandise quantity
unit_price string
MandatoryMerchandise unit price
link string
MandatoryMerchandise purchasing link. this is mandatory for open market transactions.
surcharge array * The array length is limited to three.
name string
The name of the additional amount category (e.g., discount coupon, delivery fee).
quantity string
The quantity of the additional amount category, which must be greater than zero.
unit_price string
The unit price of the additional amount category, which cannot include , and may be sent with a negative quantity
ship_to object
city string
Delivery city
country string
Delivery country. ISO3166 two digit country code.
first_name string
Recipient's first name
last_name string
Recipient's last name
phone_number string
Recipient's contact number, with the option to include the country code.
postal_code string
Postal code
state string
Delivery state. This is applicable for delivery addresses in the U.S. and Canada, Please refer to the country code.
street1 string
Provide the remaining address details accordingly
bill_to object
city string
Billing city
country string
Billing country. ISO3166 two digit country code.
first_name string
Card holder's first name
last_name string
Card holder's last name
phone_number string
Card holder's contact number. with the option to include the country code.
postal_code string
Billing postal code
state string
Billing state. This is applicable for delivery addresses in the U.S. and Canada, Please refer to the country code.
street1 string
Provide the remaining address details accordingly
settings object
display_type string
-
P
: Opens the payment page as a pop-up.
-
R
: Redirects to the payment page from the merchant's site.
autoclose string
-
Y
: Redirects to the merchant's page.
-
N
: Redirects to the payment success page (default).
call_from_app string
-
Y
: Call in the application environment (iOS, Android).
-
N
: Call in the web browser environment (default).
-
* Call the payment page within an app environment. Please refer to the External App Open in the WebView Integration Guide for further details.
call_from_scheme string
-
* Call the payment page within an app environment. Please refer to the External App Open in the WebView Integration Guide for further details.
issuer_country string
ostype string
-
P
: PC environment (default)
-
M
: Mobile environment
virtualaccount_expiry_datestring
4. Payment verification
To prevent Unauthorized modification and falsification, merchants can utilize the Payment Verification API, By sending the response parameters to the Payment Verification API, merchants can verify whether they match the fgkey received on the Eximbay server. This ensures the integrity of the transaction. For further details, please refer to the Preparation for After SDK Response section.
The parameters received from the Eximbay server via thestatus_url will be transmitted in query string format as outlined below.
currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM
After receiving the status URL and completing the transaction, please display the response code (rescode) and response message (resmsg) on the final page as follows:
• Success: rescode=0000 & resmsg=Success
• Failure: rescode=[error code] & resmsg=[error message]
The status URL output may be duplicated. Please ensure that transactions are not processed multiple times. Duplicate transactions can be identified using the transaction ID.
Response parameters received in the query string must be submitted to the Payment Verification API. Once the validation process is successfully completed by the Payment Verification API, the payment will be finalized.
curl --request POST 'https://api-test.eximbay.com/v1/payments/verify \
--header 'Authorization: Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=' \
--header 'Content-Type: application/json' \
--data '{
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
}'
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Type", "application/json");
headers.add("Authorization", "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=");
URI url = URI.create("https://api-test.eximbay.com/v1/payments/verify");
String body = "{\n" +
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
"}";
HttpEntity<String> entity = new HttpEntity<>(body, headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.POST, entity, String.class);
System.out.println(response.getBody());
<?php
$url = 'https://api-test.eximbay.com/v1/payments/verify';
$data = '{
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
}';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type: application/json', 'Authorization: Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo='));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
echo $response;
curl_close($ch);
?>
import requests
import json
url = "https://api-test.eximbay.com/v1/payments/verify"
headers = {
"Authorization": "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=",
"Content-Type": "application/json"
}
request = {
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
}
response = requests.post(url, headers=headers, data=json.dumps(request))
print(response.text)
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api-test.eximbay.com/v1/payments/verify',
'headers': {
'Authorization': 'Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=',
'Content-Type': 'application/json'
},
body: JSON.stringify({
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
})
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body);
});
string uri = "https://api-test.eximbay.com/v1/payments/verify";
string body = "{\n" +
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
"}";
WebClient webClient = new WebClient();
webClient.Headers[HttpRequestHeader.ContentType] = "application/json";
webClient.Headers[HttpRequestHeader.Authorization] = "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=";
webClient.Encoding = UTF8Encoding.UTF8;
string responseJSON = webClient.UploadString(uri, body);
Console.Write(responseJSON);
val restTemplate = RestTemplate()
val headers = HttpHeaders()
headers.add("Content-Type", "application/json")
headers.add("Authorization", "Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=")
var url = URI.create("https://api-test.eximbay.com/v1/payments/verify")
val body = "{\n" +
"data" : "currency=USD&card_number1=4111&transaction_date=20220927152250&card_number4=1111&mid=1849705C64&amount=100&access_country=KR&order_id=20220927152140&payment_method=P101&email=test@eximbay.com&ver=230&transaction_id=1849705C6420220927000016¶m3=TEST&resmsg=Success.&card_holder=TESTP&rescode=0000&auth_code=309812&fgkey=2AE38D785E05E6AF57977328908C7CD84A273B3FE6C042D537A800B0CBC783EA&transaction_type=PAYER_AUTH&pay_to=EXIMBAY.COM"
"}"
var entity = HttpEntity<String>(body, headers)
var response = restTemplate.exchange(url, HttpMethod.POST, entity, String::class.java)
println(response.body)
Response
The response code indicating a successful payment is 0000.
{
"rescode": "0000",
"resmsg": "Success",
}
5. Request for payment authorization
After completing payment authentication, invoke the Payment Authorization API to authorize the payment.
curl --request POST 'https://api-test.eximbay.com/v1/payments/confirm' \
--header 'Authorization: Basic dGVzdF8xODQ5NzA1QzY0MkMyMTdFMEIyRDo=' \
--header 'Content-Type: application/json' \
--data '{
"transaction_type" : "PAYMENT_PA",
"mid" : "1849705C64",
"payment" : {
"order_id" : "20220902101716",
"currency" : "USD",
"amount" : "1",
"payer_auth_id" : "20220902101716",
"lang" : "EN"
}
}'
미확정
미확정
미확정
미확정
미확정
미확정
Response
The response code indicating a successful payment is 0000.
{
"rescode": "0000",
"resmsg": "Success",
}